NewbeeAVR
Junior Member level 2

I wrote a inline assembly code in which port pins 0 to 5 represent PD2 to PD7 and 6 and 7 represent PB0 and PB1 respectively. High(pin) is used to make the respective pin logical HIGH. I referred avr-libc-user-manual.pdf to write the following code. But I went wrong some where.
In the inline assembly code
%A0 is reg0
%A1 is pin
%A2 is reg1
%A3 is reg2
%A4 is ddrx
%A5 is portx
I got these errors
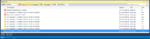
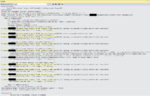
Code:
#include <avr/io.h>
class GPIO{
private:
uint8_t ddrx, portx, reg0 = 0, reg1 = 1, reg2 = 0;
void selectIO(uint8_t);
void shiftBit(uint8_t);
void readDDRx();
void readPORTx();
void writeDDRx();
void writePORTx();
void ORing();
public:
void High(uint8_t);
};
int main(void)
{
GPIO port;
port.High(0);
return 0;
}
void GPIO :: selectIO(uint8_t pin){
if((pin >= 0) && (pin <= 5)){
ddrx = 0x0A;
portx = 0x0B;
}
else if((pin >= 6) && (pin <= 7)){
ddrx = 0x04;
portx = 0x05;
}
}
//DDRx |= (1 << pin);
//PORTx |= (1 << pin);
void GPIO :: shiftBit(uint8_t pin){ //To perform (1 << pin);
asm volatile("mov %A0, %A1 \n\t"
"rjmp down \n\t"
"up: \n\t"
"clc \n\t"
"rol %A2 \n\t"
"down: \n\t"
"dec %A0 \n\t"
"brpl up \n\t"
: "+r" (reg0), "+r" (pin), "+r" (reg1) : );
}
void GPIO :: readDDRx(){
asm volatile("in %A3, %A4" : "+r" (reg2) : "I" (ddrx));
}
void GPIO :: writeDDRx(){
asm volatile("out %A3, %A4" : "+r" (reg2) : "I" (ddrx) );
}
void GPIO :: readPORTx(){
asm volatile("in %A3, %A5" : "+r" (reg2) : "I" (portx));
}
void GPIO :: writePORTx(){
asm volatile("out %A3, %A5" : "+r" (reg2) : "I" (portx) );
}
void GPIO :: ORing(){
asm volatile("or %A3, %A1" : "+r" (reg2) : "r" (reg1) );
}
void GPIO :: High(uint8_t pin){
selectIO(pin);
shiftBit(pin);
readDDRx();
ORing(); //reg2 = DDRx | (1 << pin)
writeDDRx(); //DDRx = reg2
readPORTx();
ORing(); //reg2 = PORTx | (1 << pin)
writePORTx(); //PORTx = reg2
}
In the inline assembly code
%A0 is reg0
%A1 is pin
%A2 is reg1
%A3 is reg2
%A4 is ddrx
%A5 is portx
I got these errors
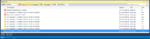
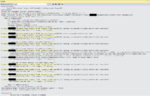