ajit_nayak87
Member level 5

I am using Mplab x Ide with v3.61 on Xc8 compiler, PIC18F24K40. I used MCC generated UART code for send and receive data. For understanding I am clubling my code and posting part of it.
My main code look like this.
And tested Serial communication. with this code i could print welcome on Serial Monitor
The problem i am facing is. When i try to send data 0103000000Ac5CD In ASCii format i receice as charcter ,but when i try to send in hex format i could not able to receive nothing. If am printing data like i used in working hello print example i got garbage value.
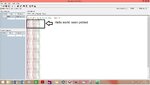
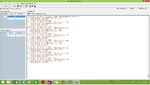
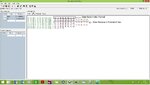
my questions:
1) how to receive data from uart as string
2) how to receive hex value.
My main code look like this.
Code:
#include "mcc_generated_files/mcc.h"
#define EUSART_TX_BUFFER_SIZE 8
#define EUSART_RX_BUFFER_SIZE 8
/**
Section: Global Variables
*/
volatile uint8_t eusartTxHead = 0;
volatile uint8_t eusartTxTail = 0;
volatile uint8_t eusartTxBuffer[EUSART_TX_BUFFER_SIZE];
volatile uint8_t eusartTxBufferRemaining;
volatile uint8_t eusartRxHead = 0;
volatile uint8_t eusartRxTail = 0;
volatile uint8_t eusartRxBuffer[EUSART_RX_BUFFER_SIZE];
volatile uint8_t eusartRxCount;
#define LED_RX RC7 // Pin assigned RX LED
#define LED_TX RC6 // Pin assigned TX LED
#define LED RC2 // Pin assigned for LED
#define DE RC5
unsigned int count=0;
char data=0;
static int DEVICE_ID=1;
char rxbuf[50]=" ";
unsigned char Function_code=0X03;
static int index=0;
static int rec_flag = 0;
static int check_flag = 1;
unsigned char buff[10];
char data1[10];
unsigned char buf[20]; //array to hold twenty bytes
void EUSART_Initialize(void)
{
// disable interrupts before changing states
PIE3bits.RCIE = 0;
PIE3bits.TXIE = 0;
// Set the EUSART module to the options selected in the user interface.
// ABDOVF no_overflow; SCKP Non-Inverted; BRG16 16bit_generator; WUE disabled; ABDEN disabled;
BAUD1CON = 0x08;
// SPEN enabled; RX9 8-bit; CREN enabled; ADDEN disabled; SREN enabled;
RC1STA = 0xB0;
// TX9 8-bit; TX9D 0; SENDB sync_break_complete; TXEN enabled; SYNC asynchronous; BRGH hi_speed; CSRC master;
TX1STA = 0xA4;
TRISCbits.TRISC7 = 1; //As Prescribed in Datasheet
TRISCbits.TRISC6 = 1;
RC1STAbits.CREN=1;
TX1STAbits.TXEN=1;
// Baud Rate = 9600; SP1BRGL 207;
SP1BRGL = 0xCF;
// Baud Rate = 9600; SP1BRGH 0;
SP1BRGH = 0x00;
// initializing the driver state
eusartTxHead = 0;
eusartTxTail = 0;
eusartTxBufferRemaining = sizeof(eusartTxBuffer);
eusartRxHead = 0;
eusartRxTail = 0;
eusartRxCount = 0;
// enable receive interrupt
PIE3bits.RCIE = 1;
}
uint8_t EUSART_Read(void)
{
uint8_t readValue = 0;
while(0 == eusartRxCount)
{
}
readValue = eusartRxBuffer[eusartRxTail++];
if(sizeof(eusartRxBuffer) <= eusartRxTail)
{
eusartRxTail = 0;
}
PIE3bits.RCIE = 0;
eusartRxCount--;
PIE3bits.RCIE = 1;
eusartRxBuffer[8];
return readValue;
}
void EUSART_Write(uint8_t txData)
{
while(0 == eusartTxBufferRemaining)
{
}
if(0 == PIE3bits.TXIE)
{
TX1REG = txData;
}
else
{
PIE3bits.TXIE = 0;
eusartTxBuffer[eusartTxHead++] = txData;
if(sizeof(eusartTxBuffer) <= eusartTxHead)
{
eusartTxHead = 0;
}
eusartTxBufferRemaining--;
}
PIE3bits.TXIE = 1;
}
char getch(void)
{
return EUSART_Read();
}
void putch(char txData)
{
EUSART_Write(txData);
}
void EUSART_Transmit_ISR(void)
{
// add your EUSART interrupt custom code
if(sizeof(eusartTxBuffer) > eusartTxBufferRemaining)
{
TX1REG = eusartTxBuffer[eusartTxTail++];
if(sizeof(eusartTxBuffer) <= eusartTxTail)
{
eusartTxTail = 0;
}
eusartTxBufferRemaining++;
}
else
{
PIE3bits.TXIE = 0;
}
}
void EUSART_Receive_ISR(void)
{
if(1 == RC1STAbits.OERR)
{
// EUSART error - restart
RC1STAbits.CREN = 0;
RC1STAbits.CREN = 1;
}
// buffer overruns are ignored
eusartRxBuffer[eusartRxHead++] = RC1REG;
if(sizeof(eusartRxBuffer) <= eusartRxHead)
{
eusartRxHead = 0;
}
eusartRxCount++;
}
/* Send Data Serially */
void send_string(const char *x)
{
while(*x)
{
EUSART_Write(*x++);
}
}
/* Timer Interrupt Service Routine Program*/
void Blink_Count()
{
if(PIR0bits.TMR0IF == 1)
{
PIR0bits.TMR0IF =0;
count=count+1;
if(count>=15)
{
// LED=!LED;
count=0;
}
}
}
void main(void)
{
while (1)
{
data= EUSART_Read();
rxbuf[10]=data;
printf("%s\n",&rxbuf[10]);
__delay_ms(150);
}
}
Code:
void main(void)
{
EUSART_Initialize();
while (1)
{
char array[20] = "Hello World";
printf("%s\n",array);
__delay_ms(150);
}
}
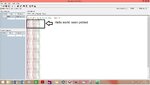
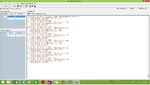
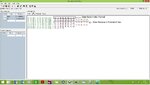
my questions:
1) how to receive data from uart as string
2) how to receive hex value.