lulezo
Junior Member level 3

Hi guys
I am trying to interface the 4*4 matrix keyboard with lpc2148, I wrote the code for that, and to display the key pressed in the lcd, but it didn't work.
The keyboard connections:
columns: column 1 to column 4 connected to P1.21 to P1.24
rows: row 1 to row 4 connected to P1.17 to P1.20
LCD connections:
the 4-bit data: from P0.19 to P0.22
Enable: P0.10
R/W: P0.12
RS: P0.13
Here is the code:
I am trying to interface the 4*4 matrix keyboard with lpc2148, I wrote the code for that, and to display the key pressed in the lcd, but it didn't work.
The keyboard connections:
columns: column 1 to column 4 connected to P1.21 to P1.24
rows: row 1 to row 4 connected to P1.17 to P1.20
LCD connections:
the 4-bit data: from P0.19 to P0.22
Enable: P0.10
R/W: P0.12
RS: P0.13
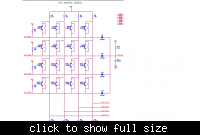
Here is the code:
Code:
#include <LPC214X.H>
void init_keyb(void);
void init_lcd(void);
unsigned char read_key(void);
unsigned char decode(int, int);
int get_row(int);
int get_col(int);
unsigned char mat(int, int);
void cmd(unsigned char);
void datal(unsigned char);
void delay(int);
int main ()
{
unsigned char f=0xFF; //for unpressed key
init_keyb();
init_lcd();
while (1)
{
f = read_key();
if (f!=0xFF) //if no key is pressed then continue polling
datal(f);
else
continue;
}
}
void init_keyb(void)
{
IODIR1 = 0x01E00000; //making rows as I/P and columns as O/P
}
void init_lcd(void)
{
IODIR0 = 0x00783400;
cmd(0x28);
cmd(0x01);
cmd(0x02);
cmd(0x06);
cmd(0x0C);
}
unsigned char read_key()
{
int row=0,col=0,i;
unsigned char f=0xFF;
IODIR1 = 0x01E00000; //making rows as I/P and columns as O/P
row = IOPIN1 & 0x001E0000;
IODIR1 = 0x001E0000; //making rows as O/P and columns as I/P
col = IOPIN1 & 0x01E00000;
IODIR1 = 0x01E00000; //making rows as I/P and columns as O/P
f = decode(row,col);
return (f);
}
unsigned char decode(int row, int col)
{
int r=0,c=0;
unsigned char f=0xFF;
r=get_row(row);
c=get_col(col);
if (r!=0xFF && c!=0xFF)
f=mat(r,c);
else
f=0xFF;
return (f);
}
unsigned char mat(int r, int c)
{
unsigned char m[4][4]={{'A','B','C','D'},
{'E','F','G','H'},
{'I','J','K','L'},
{'M','N','O','P'},
};
unsigned char f;
f=m[r][c];
return (f);
}
int get_row(int row)
{
int r=0xff;
switch (row ^ 0x001E0000)
{
case 0x00020000:
r=0;
break;
case 0x00040000:
r=1;
break;
case 0x00080000:
r=2;
break;
case 0x00100000:
r=3;
break;
default:
break;
}
return (r);
}
int get_col (int col)
{
int c=0xff;
switch (col ^ 0x01E00000)
{
case 0x00200000:
c=0;
break;
case 0x00400000:
c=1;
break;
case 0x00800000:
c=2;
break;
case 0x01000000:
c=3;
break;
default:
break;
}
return (c);
}
void cmd(unsigned char com)
{
int a=0;
a=com&0xF0;
a=a<<15;
IOCLR0 = 0x00783400;
IOSET0 = 0x00000400;
IOSET0 = a;
delay(10000);
IOCLR0 = 0x00000400;
a=com&0x0F;
a=a<<19;
IOCLR0 = 0x00783400;
IOSET0 = 0x00000400;
IOSET0 = a;
delay(10000);
IOCLR0 = 0x00000400;
}
void datal(unsigned char d)
{
int b=0;
b=d&0xF0;
b=b<<15;
IOCLR0 = 0x00783400;
IOSET0 = 0x00002400;
IOSET0 = b;
delay(10000);
IOCLR0 = 0x00000400;
b=d&0x0F;
b=b<<19;
IOCLR0 = 0x00783400;
IOSET0 = 0x00002400;
IOSET0 = b;
delay(10000);
IOCLR0 = 0x00000400;
}
void delay(int count)
{
int i=0,j=0;
for (i=0;i<count;i++)
for (j=0;j<35;j++);
}
Last edited by a moderator: