Athul
Newbie

Hello,
I'm completely new SPI protocol. I wrote my first code today. It was uisng polling method from these two tutorials
https://sites.google.com/site/qeewiki/books/avr-guide/spi
https://maxembedded.com/2013/11/the-spi-of-the-avr/
First link also has interrupt based code. But it's not working.
Can anyone suggest me how to properly code this.
I don't have two Arduino, so I'm using Protues to simulate code for ATMega168
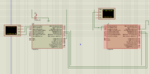
Thanks
I'm completely new SPI protocol. I wrote my first code today. It was uisng polling method from these two tutorials
https://sites.google.com/site/qeewiki/books/avr-guide/spi
https://maxembedded.com/2013/11/the-spi-of-the-avr/
First link also has interrupt based code. But it's not working.
Code C++ - [expand] 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 // this program enables SPI communication and // Sets the AVR into Master mode using interrupts #include <avr/io.h> #include <avr/interrupt.h> #include <util//delay.h> volatile uint8_t data; int main (void) { char blah = 'B'; DDRB |= (1<<2)|(1<<3)|(1<<5); // SCK, MOSI and SS as outputs DDRB &= ~(1<<4); // MISO as input SPCR |= (1<<MSTR); // Set as Master SPCR |= (1<<SPR0)|(1<<SPR1); // divided clock by 128 SPCR |= (1<<SPIE); // Enable SPI Interrupt SPCR |= (1<<SPE); // Enable SPI sei(); SPDR = 'A'; while(!(SPSR & (1<<SPIF))); while(1) { if ((SPSR & (1<<SPIF)) > 1) // checks to see if the SPI bit is clear data=blah; // send the data // if you use multiple slaves, switch slave here. } } ISR (SPI_STC_vect) { SPDR = data; _delay_ms(100); }
Code C++ - [expand] 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 // this program enables SPI communication and // Sets the AVR into Slave mode #include <avr/io.h> #include <avr/interrupt.h> #include <util/delay.h> //#include "USART.h" volatile uint8_t data; int main (void) { DDRB &= ~((1<<2)|(1<<3)|(1<<5)); // SCK, MOSI and SS as inputs DDRB |= (1<<4); // MISO as output DDRC = 0XFF; SPCR &= ~(1<<MSTR); // Set as slave SPCR |= (1<<SPR0)|(1<<SPR1); // divide clock by 128 SPCR |= (1<<SPIE); // Enable SPI Interrupt SPCR |= (1<<SPE); // Enable SPI sei(); while(1) { _delay_ms(10); } } ISR (SPI_STC_vect) { data = SPDR; transmitByte(data); }
Can anyone suggest me how to properly code this.
I don't have two Arduino, so I'm using Protues to simulate code for ATMega168
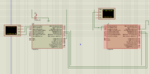
Thanks