BiNa2605
Full Member level 3

- Joined
- Sep 1, 2015
- Messages
- 179
- Helped
- 6
- Reputation
- 12
- Reaction score
- 4
- Trophy points
- 18
- Location
- VietNam
- Activity points
- 1,357
Hi everyone,
I am working with LIS3DH and have some problems relating to getting data from XYZ axis. In LIS3DH (accelerometer from ST), I enabled FIFO function and operate in stream mode. I use visual C# to display data for testing and debugging. My problem is the data I read it is still one value and does not change even though I move the accelerometer sensor.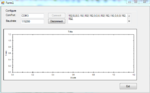
And this all of my code.
I am working with LIS3DH and have some problems relating to getting data from XYZ axis. In LIS3DH (accelerometer from ST), I enabled FIFO function and operate in stream mode. I use visual C# to display data for testing and debugging. My problem is the data I read it is still one value and does not change even though I move the accelerometer sensor.
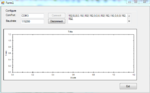
And this all of my code.
Code:
/******************************************************************************/
//This codes are used for communicate with Accelerometer sensor LISDH 3-axis
//Write and Read data from Accelerometer sensor
//Author: Dang Trung Nam
//Date: 2016 - Jan - 21
//Hardware: PIC16F1513 Extreme Low Power Consumption (MCU)- LIS3DH (Accelerometer)
//Software: MikroC Pro for PIC
//SPI interface
/******************************************************************************/
#define CS PORTC.B1 //Chip Select on PORTC.B1
#define WRS 0x00 //Written into the device (sensor) and the address remains unchanged
#define WRI 0x40 //Written into the device (sensor) and the address is auto incremented
#define RES 0x80 //The device (sensor) is read and the address remains unchanged
#define REI 0xC0 //The device (sensor) is read and the address is auto incremented
//Declare Global variables
int iXYZ[6], isample; //Store 3-axis value
int iX[2],iY[2],iZ[2];
unsigned char txt[6]="123";
unsigned char _data = 0x30;
//Declare sub-functions
void InitGPIO(); //Initiate GPIO and SPI interface
unsigned char ISenID(); //Determine Accelerometer Sensor
void InitLIS3DH(); //Initiate Sensor mode and operation
unsigned char OVRN_StreamMode();
void Read_XYZ(); //SPI Read function
void Read_X(int);
void Read_Y(int);
void Read_Z(int);
void ToggleLed();
void main() {
unsigned char ucSensor,ucOVRN;
int j;
short sTest,sXTest;
char txt[9];
char ctest;
InitGPIO(); //Initiate GPIO and SPI interface
InitLIS3DH(); //Initiate Sensor mode
CS = 1; //Stop all SPI
while(1)
{
ucSensor = ISenID();
if(ucSensor == 0x33)
{
Read_XYZ();
while((ucOVRN = OVRN_StreamMode())&0x02);
/*
for(j = 0; j < 6; j++)
{
UART1_Write(iXYZ[j]);
delay_ms(100);
}
*/
for(j = 0; j<32; j++)
{
Read_X(j);
Read_Y(j);
Read_Z(j);
UART1_Write(iX[1]);
delay_ms(100);
UART1_Write(iX[0]);
delay_ms(100);
UART1_Write(iY[1]);
delay_ms(100);
UART1_Write(iY[0]);
delay_ms(100);
UART1_Write(iZ[1]);
delay_ms(100);
UART1_Write(iZ[0]);
}
ToggleLed();
}
else {PORTB.B4 = 0;}
}
}
//Initiate GPIO and SPI interface
void InitGPIO(){
OSCCON = 0b01101010; //Internal oscillator clock 4MHz
ANSELC = 0;
ANSELB = 0;
TRISB.B4 = 0; //PORTB pin4 output --- Test LED
PORTB.B4 = 1; //Led off
TRISC.B1 = 0; //PORTC pin1 output --- Chip select
//Master Mode - clock FOSC/4 (4Mhz/4) - clock idle state low
//Data transmitted on low to high edge
//Input data sampled at the middle of internal
//SPI1_Init(); //Initiate SPI interface
SPI1_Init_Advanced(_SPI_MASTER_OSC_DIV4, _SPI_DATA_SAMPLE_MIDDLE, _SPI_CLK_IDLE_LOW, _SPI_HIGH_2_LOW);
UART1_Init(115200);
delay_ms(500); //Wait for UART module stablize
}
//Initiate Sensor
//Mode: Normal --- Output Data Rate (ODR) = 50Hz
void InitLIS3DH(){
//Enable ADC block
CS = 0; //Enable SPI
delay_ms(1);
SPI1_Write(WRS | 0x1F); //I want to setup (write) in the TEMP_CFG_REG register
SPI1_Write(0x80); //ADC enable
delay_ms(1);
CS = 1;
delay_ms(1);
//Setup Operation for sensor
CS = 0; //Enable SPI
delay_ms(1);
SPI1_Write(WRS | 0x20); //I want to setup (write) in the CTRL_REG1 register
SPI1_Write(0x47); //Data rate 50Hz, normal mode, 3-axis enable
delay_ms(1);
CS = 1;
delay_ms(1);
CS = 0; //Enable SPI
delay_ms(1);
SPI1_Write(WRS | 0x21); //CTRL_REG2 register
SPI1_Write(0b00000000); //Don't use High Pass Filter
delay_ms(1);
CS = 1; //Disable SPI
delay_ms(1);
CS = 0; //Enable SPI
delay_ms(1);
SPI1_Write(WRS | 0x22); //CTRL_REG3 register
SPI1_Write(0b00000010); //FIFO overrun interrupt.
delay_ms(1);
CS = 1; //Disable SPI
delay_ms(1);
//Normal mode, high resolution
//Scale selection: 2G
//Block continous update
//SPI 4 wire interface CS, SPC, SDI, SDA
//Self test disable
CS = 0; //Enable SPI
delay_ms(1);
SPI1_Write(WRS | 0x23); //CTRL_REG4 register
SPI1_Write(0b00001000); //Described above HR = 1 - high resolution
delay_ms(1);
CS = 1; //Disable SPI
delay_ms(1);
//Enable FIFO
CS = 0;
delay_ms(1);
SPI1_Write(WRS | 0x24);
SPI1_Write(0x40); //Enable FIFO
delay_ms(1);
CS = 1;
delay_ms(1);
//Enable FIFO Mode for measure X,Y,Z data
//Trigger event liked to trigger signal on INT1
CS = 0;
delay_ms(1);
SPI1_Write(WRS | 0x2E);
SPI1_Write(0x80); //Enable stream Mode
delay_ms(1);
CS = 1;
delay_ms(1);
//Configure INT1_CFG
CS = 0;
delay_ms(1);
SPI1_Write(WRS | 0x30);
SPI1_Write(0x00); //Default Direction
delay_ms(1);
CS = 1;
}
//Read Address of Accelerometer at WHO_AM_I register 0x0F
unsigned char ISenID(){
unsigned char ucID;
//ucTemp = RES | 0x0F; //ucTemp = 0x8F;
CS = 0; //Pull-down CS pin to enable SPI
delay_ms(1); //delay for setup time
//Send the SPI1_Write function with the aim
//I want to Read data from 0x0F register
SPI1_Write(RES | 0x0F);
//Read value from 0x8F register and store in ucID variable
ucID = SPI1_Read(0);
delay_ms(1); //delay for holding time
CS = 1; //Pull-up CS pin to disable SPI
return ucID;
//Default value of WHO_AM_I value is 0x33
//Run the while loop until satisfy the condition ucID = 0x33
//That is the expected sensor
}
unsigned char OVRN_StreamMode()
{
unsigned char ucFlag;
CS = 0; //Enable SPI
delay_ms(1);
SPI1_Write(RES | 0x22);
ucFlag = SPI1_Read(0);
delay_ms(1);
CS = 1; //Disable SPI
return ucFlag;
}
//Read 3-axis value
void Read_XYZ(){
unsigned char ucstt;
unsigned int i;
//Read Status Register STATUS_REG 0x27
do{
CS = 0; //Enable SPI
delay_ms(1);
SPI1_Write(RES | 0x27); //Send I want to read Status register
ucstt = SPI1_Read(0); //Read value from STATUS_AUX register
delay_ms(1);
CS = 1; //Disable SPI
delay_ms(1);
}while(ucstt & 0x08); //Stop the while loop when STATUS_REG[3] = 1
//1: a new set of data is available
/*
//Checking whether data has overwritten or not. This using for interrupt_function
if((ucst & 0x80) == 0x80) //A new set of data has over written the previous ones
{
//Using LED to notify that
PORTB.B4 = 0;
delay_ms(500);
PORTB.B4 = 1;
delay_ms(500);
}
*/
//Read XYZ value (read continously: Address will be incremented)
ucstt = 0; //Clear value
/*
CS = 0; //Enable SPI
delay_ms(1);
SPI1_Write(REI | 0x28); //0xE8
for(i = 0; i < 6; i++ ){
iXYZ[i] = 0;
iXYZ[i] = SPI1_Read(0);
delay_us(200);
}
delay_ms(1);
CS = 1; //Disable SPI
*/
}
void Read_X(int index)
{
//Read OUTX_H
CS = 0;
delay_ms(1);
SPI1_Write(RES | 0x29);
iX[1] = 0;
iX[1] = SPI1_Read(index);
delay_ms(1);
CS = 1;
delay_ms(1);
//Read OUTX_L
CS = 0;
delay_ms(1);
SPI1_Write(RES | 0x28);
iX[0] = 0;
iX[0] = SPI1_Read(index);
delay_ms(1);
CS = 1;
}
void Read_Y(int index)
{
//Read OUTY_H
CS = 0;
delay_ms(1);
SPI1_Write(RES | 0x2B);
iY[1] = 0;
iY[1] = SPI1_Read(index);
delay_ms(1);
CS = 1;
delay_ms(1);
//Read OUTY_L
CS = 0;
delay_ms(1);
SPI1_Write(RES | 0x2A);
iY[0] = 0;
iY[0] = SPI1_Read(index);
delay_ms(1);
CS = 1;
}
void Read_Z(int index)
{
//Read OUTZ_H
CS = 0;
delay_ms(1);
SPI1_Write(RES | 0x2D);
iZ[1] = 0;
iZ[1] = SPI1_Read(index);
delay_ms(1);
CS = 1;
delay_ms(1);
//Read OUTZ_L
CS = 0;
delay_ms(1);
SPI1_Write(RES | 0x2C);
iZ[0] = 0;
iZ[0] = SPI1_Read(index);
delay_ms(1);
CS = 1;
}
void ToggleLed()
{
PORTB.B4 = 0;
delay_ms(200);
PORTB.B4 = 1;
delay_ms(200);
}