apoorv20
Newbie level 2

Re: AD7730 problems
Hi All
I'm trying to run AD7730 with Atmega16 but there is some problem with the SPI Interface. I'm trying to write and read the DAC register to check if communication is proper.
Please verify the attached schematic and code.
Thanks in advance.
Regards
Apoorva Gag
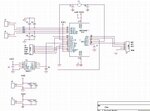
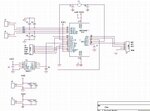
Hi All
I'm trying to run AD7730 with Atmega16 but there is some problem with the SPI Interface. I'm trying to write and read the DAC register to check if communication is proper.
Please verify the attached schematic and code.
Thanks in advance.
Regards
Apoorva Gag
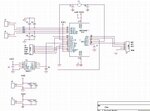
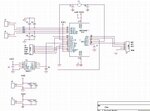
Code:
#include <avr/io.h>
#include <avr/interrupt.h>
#include <avr/pgmspace.h>
#include <math.h>
#include <inttypes.h>
#include <util/delay.h>
#include <string.h>
#include <stdlib.h>
#include <avr/signal.h>
#include "SPI_routines.h"
#include "UART_routines.h"
#define ADE_ASSERT() PORTB |= 0x10;
#define ADE_DEASSERT() PORTB &= 0xEF;
#define STATUS 0
#define DATA 1
#define MODE 2
#define FILTER 3
#define DAC 4
#define OFFSET 5
#define GAIN 6
#define TEST 7
char Print_Data[10];
unsigned int count;
int main(void){
unsigned int read_data,count;
unsigned int a,i;
DDRA = 255;
DDRB = 255;
PORTB = 0x00; // ADE7753 Reset pin set low
// Initializations
uart0_init();
spi_init();
// ADE7753 Reset and CS ON
ADE_ASSERT()
PORTB = 0x08; // ADE7753 Reset pin pulled high
_delay_ms(100);
ADE_DEASSERT() // ADE7753 CS pin pulled low
_delay_ms(100);
while(1)
{
PORTA = 0x00;
_delay_ms(200);
_delay_us(200);
PORTA = 0x80; //Check LED at PORTA
_delay_ms(200);
_delay_ms(200);
SPI_transmit(0x04); //Send DAC Write Command - Write to the communication Register
_delay_us(1);
SPI_transmit(0x10); //Write 16 to the DAC Register
_delay_us(1);
SPI_transmit(0x14); //Send DAC Read Command - Write to the communication Register
_delay_us(1);
count = SPI_receive(); //Read DAC Register
itoa(count,Print_Data,10);//Print Count value, if 16 then SPI working properly
transmitString(Print_Data);
transmitString("\t");
transmitString("CBC \r\n");
}
}
Last edited: