Paritoshgiri
Member level 5

Hi
I am using PIC 18F4550 for ADC. I am using an input voltage range from 1 to 4.5 V and I am getting the digital data in hyperterminal of my PC. I can successfully get a corresponding digital signal of an analog input but when the analog input value changes fast, many values are missed when I see the digital output on hyperterminal. So I need to increase the sampling rate of ADC so that I can see all the values in hyperterminal. I need a sampling rate of atleast 1 KHz. How can I increase the sampling rate so that I can see all the digital output of corresponding analog inputs in a hyperterminal? Please help me with this.
My working code:
The schematics of my circuit:
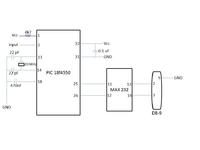
I am using PIC 18F4550 for ADC. I am using an input voltage range from 1 to 4.5 V and I am getting the digital data in hyperterminal of my PC. I can successfully get a corresponding digital signal of an analog input but when the analog input value changes fast, many values are missed when I see the digital output on hyperterminal. So I need to increase the sampling rate of ADC so that I can see all the values in hyperterminal. I need a sampling rate of atleast 1 KHz. How can I increase the sampling rate so that I can see all the digital output of corresponding analog inputs in a hyperterminal? Please help me with this.
My working code:
Code:
#include <p18f4550.h>
#include <delays.h>
void Read_ADC(void);
void Send_Value (void);
unsigned int adc;
unsigned float result;
unsigned int voltage;
void main(void)
{
TRISA = 0b00000001;
ADCON0 = 0b00000001;
// A/D is ON
ADCON1 = 0b00001110;
// Vref- set to Vss
// Vref+ set to Vdd
// AN0 is analog - others are digital
ADCON2 = 0b10111111;
// right justify
// Acq time 20TAD
// clk Frc
TXSTA = 0b10100010;
// 8-bit
// async
// low speed (BRGH=0)
RCSTA = 0b10010000;
SPBRG = 77;
while (1)
{
Read_ADC();
Send_Value();
Delay10KTCYx(240); //Delay 200 ms
Delay10KTCYx(240); //Delay 200 ms
}
}
void Read_ADC (void)
{
voltage = 0; //globally defined as unsigned long
Delay10TCYx(24); // Delay for 240TCY allow sampling cap to charge
ADCON0bits.GO = 1; //start converting
while (ADCON0bits.DONE == 1); //wait for EOC
voltage = ((unsigned int)ADRESH << 8) | ADRESL; //combines two bytes into a long int
result = (float)(voltage * 5)/1023;
adc = result * 10000;
//voltage = voltage * 5000; //Vref is in milliVolts
//voltage = voltage/1023; //10 bit ADC
}
void Send_Value (void)
{
char ch;
ch = (adc/10000) % 10; //extract thousands digit
ch = ch + 48; // now it is in ASCII
while (PIR1bits.TXIF == 0);
{
TXREG = ch;
}
while (PIR1bits.TXIF == 0);
TXREG = '.'; //We need a decimal point
ch = (adc/1000) % 10; //extract thousands digit
ch = ch + 48; // now it is in ASCII
while (PIR1bits.TXIF == 0);
{
TXREG = ch;
}
ch = (adc/100) % 10; //extract hundredth digit
ch = ch + 48;
while (PIR1bits.TXIF == 0);
TXREG = ch;
ch = (adc/10) % 10; //extract tenth digit
ch = ch + 48;
while (PIR1bits.TXIF == 0);
TXREG = ch;
ch = adc % 10;
ch = ch + 48;
while (PIR1bits.TXIF == 0);
TXREG = ch;
while (PIR1bits.TXIF == 0);
TXREG = '\n';
while (PIR1bits.TXIF == 0);
TXREG = '\r';
while (PIR1bits.TXIF == 0);
}
The schematics of my circuit:
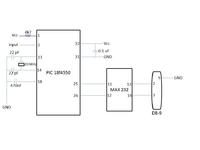